I am working with Google Ads Scripts. Based on my use case, I need to get the impressions, clicks, and other statistics about the products I have in my Google Ads account.
These product statistics will be analyzed in the script and based on certain criteria a Google Sheet document will be updated. The script goes like, retrieving all products (along with their statistics) from Google Ads account, see if the products impression and clicks meet a category (30 Impression, 1 click = Category "A", 20 Impressions, 0 Clicks = "Category B"), determines the product category, open Google Sheet hosted on Google Drive and updates the Category column in the Sheet based on product name or id.
Currently, I am facing an issue with retrieving Products and their statistics
from Google Ads Account
using Ads Script
. I used the following code snippets to look for products but found no method or class that would help me achieve my desired results. I can only retrieve the productGroups, AdGroups, and Campaigns
but none of them allow me to extract individual products.
function productGroup(){ var prodGroupIter = AdsApp.productGroups().get(); while (prodGroupIter.hasNext()){ Logger.log("prodGroup: ") var prodGroup = prodGroupIter.next(); Logger.log(prodGroup.getValue()); } } function adGroup() { var adGroupIterator = AdsApp.adGroups().get(); while (adGroupIterator.hasNext()) { var adGroup = adGroupIterator.next(); Logger.log("adGroup: ") Logger.log(adGroup.getName()); } } function campaign(){ var campIter = AdsApp.campaigns().get(); while (campIter.hasNext()){ var ads = campIter.next().ads().get(); while(ads.hasNext()){ Logger.log(ads.next().getStatsFor("LAST_30_DAYS")); } } } function sheet() { var sheetURL = "https://docs.google.com/spreadsheets/d/1W0zhRrQa1P7qjQf0eXXw-QzdcPKAlDPiVBOcIVEfDgw/edit#gid=1428103052"; var sheet = SpreadsheetApp.openByUrl(sheetURL); } function main(){ campaign(); adGroup(); productGroup(); }
I then reached out to the Ads Script support team and found out that it is not possible. But they suggested that I can use Shopping Performance Report, Product Partition Report, shopping_performance_view or product_group_view. They are part of AdWord API, and I do not know how to work with them.
So, I am looking for a Google Ads Script
that would help me get a list of products (with detailed statistics) from Googe Ads and I am stuck with how to use the above-mentioned AdWord
reporting endpoints to do it.
Here is the list of products in my Google Ads Account. They are 107 products along with their statistics. 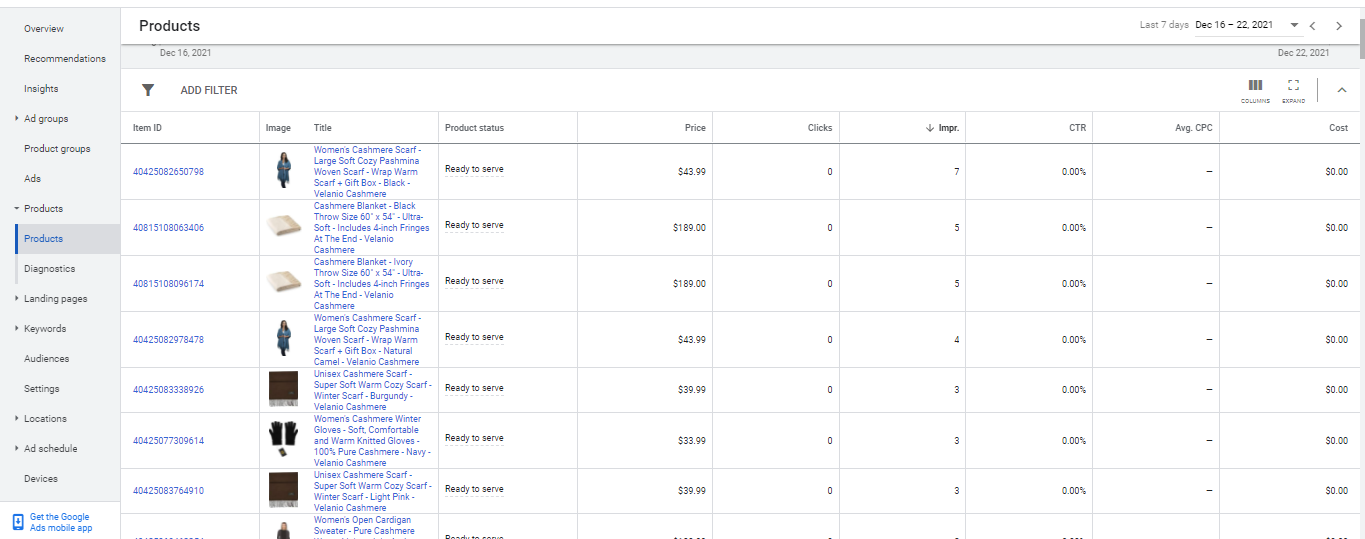
Please, help with at least comments, ideas, and suggestions, I can write code but I am not sure what I am missing on