I have a problem with my nodejs code and the connection to the official whatsapp business api.
The bot connects the webhook correctly, the messages arrive to the server correctly but the code I have implemented to make it respond is not being effective, I checked the code from top to bottom but I can't find the fault.
I leave you the codes so you have more context:
whatsappController.js:
const fs = require("fs"); const myConsole = new console.Console(fs.createWriteStream("./logs.txt")); const whatsappService = require("../services/whatsappService") const VerifyToken = (req, res) => { try { var accessToken = "456E7GR****************************"; var token = req.query["hub.verify_token"]; var challenge = req.query["hub.challenge"]; if(challenge != null && token != null && token == accessToken){ res.send(challenge); } else{ res.status(400).send(); } } catch(e) { res.status(400).send(); } } const ReceivedMessage = (req, res) => { try { var entry = (req.body["entry"])[0]; var changes = (entry["changes"])[0]; var value = changes["value"]; var messageObject = value["messages"]; if(typeof messageObject != "undefined"){ var messages = messageObject[0]; var text = GetTextUser(messages); var number = messages["from"]; myConsole.log("Message: " + text + " from: " + number); whatsappService.SendMessageWhatsApp("The user say: " + text, number); myConsole.log(messages); myConsole.log(messageObject); } res.send("EVENT_RECEIVED"); }catch(e) { myConsole.log(e); res.send("EVENT_RECEIVED"); } } function GetTextUser(messages){ var text = ""; var typeMessage = messages["type"]; if(typeMessage == "text"){ text = (messages["text"])["body"]; } else if(typeMessage == "interactive"){ var interactiveObject = messages["interactive"]; var typeInteractive = interactiveObject["type"]; if(typeInteractive == "button_reply"){ text = (interactiveObject["button_reply"])["title"]; } else if(typeInteractive == "list_reply"){ text = (interactiveObject["list_reply"])["title"]; }else{ myConsole.log("sin mensaje"); } }else{ myConsole.log("sin mensaje"); } return text; } module.exports = { VerifyToken, ReceivedMessage }
The second file is whatsappService which I make the connection with the api using the token and I also send the format of the message I want to send when I receive a hello for example...
const https = require("https"); function SendMessageWhatsApp(textResponse, number){ const data = JSON.stringify({ "messaging_product": "whatsapp", "recipient_type": "individual", "to": number, "type": "text", "text": { "preview_url": false, "body": textResponse } }); const options = { host:"graph.facebook.com", path:"/v15.0/1119744*************/messages", method:"POST", body:data, headers: { "Content-Type":"application/json", Authorization:"Bearer EAAWNbICfuWEBAK5ObPbD******************************************************" } }; const req = https.request(options, res => { res.on("data", d=> { process.stdout.write(d); }); }); req.on("error", error => { console.error(error); }); req.write(data); req.end(); } module.exports = { SendMessageWhatsApp };
Then I declare the routes for the get (to check token) and post (to receive and reply to messages) methods:
const expres = require("express"); const router = expres.Router(); const whatsappController = require("../controllers/whatsappControllers"); router .get("/", whatsappController.VerifyToken) .post("/", whatsappController.ReceivedMessage) module.exports = router;
Last but not least the index file for the code to run correctly:
const express = require("express"); const apiRoute = require("./routes/routes"); const app = express(); const PORT = process.env.PORT || 3000 app.use(express.json()); app.use("/whatsapp", apiRoute); app.listen(PORT, () => (console.log("El puerto es: " + PORT)));
I should clarify that I did the tests with Postman and they were all successful, it responds and receives messages correctly, finally I did the tests by uploading the bot to the Azure service and it works without problem until it has to answer/replicate the user's message.
The bot is not responding to the user when he talks to it but everything arrives correctly to the server and it processes it with a 200 response. I attach the evidence that there is no problem in the reception.
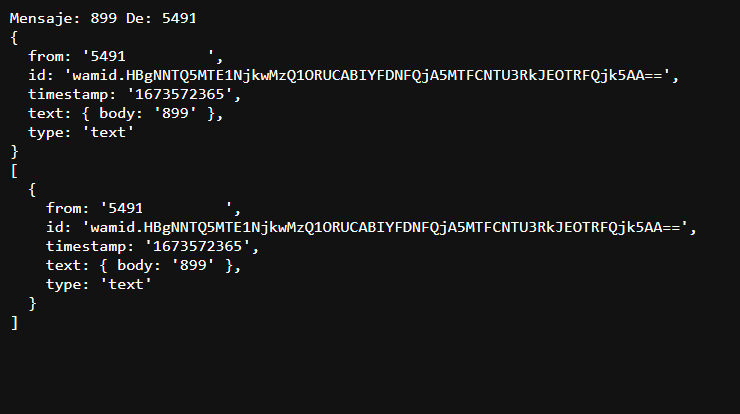
Finally I must say that in the meta platform I have everything configured as specified by the same platform, I have already configured the api to answer the messages through the webhooks and everything is correct, I just can't get the bot to answer correctly.
The bot is hosted in the Azure service.
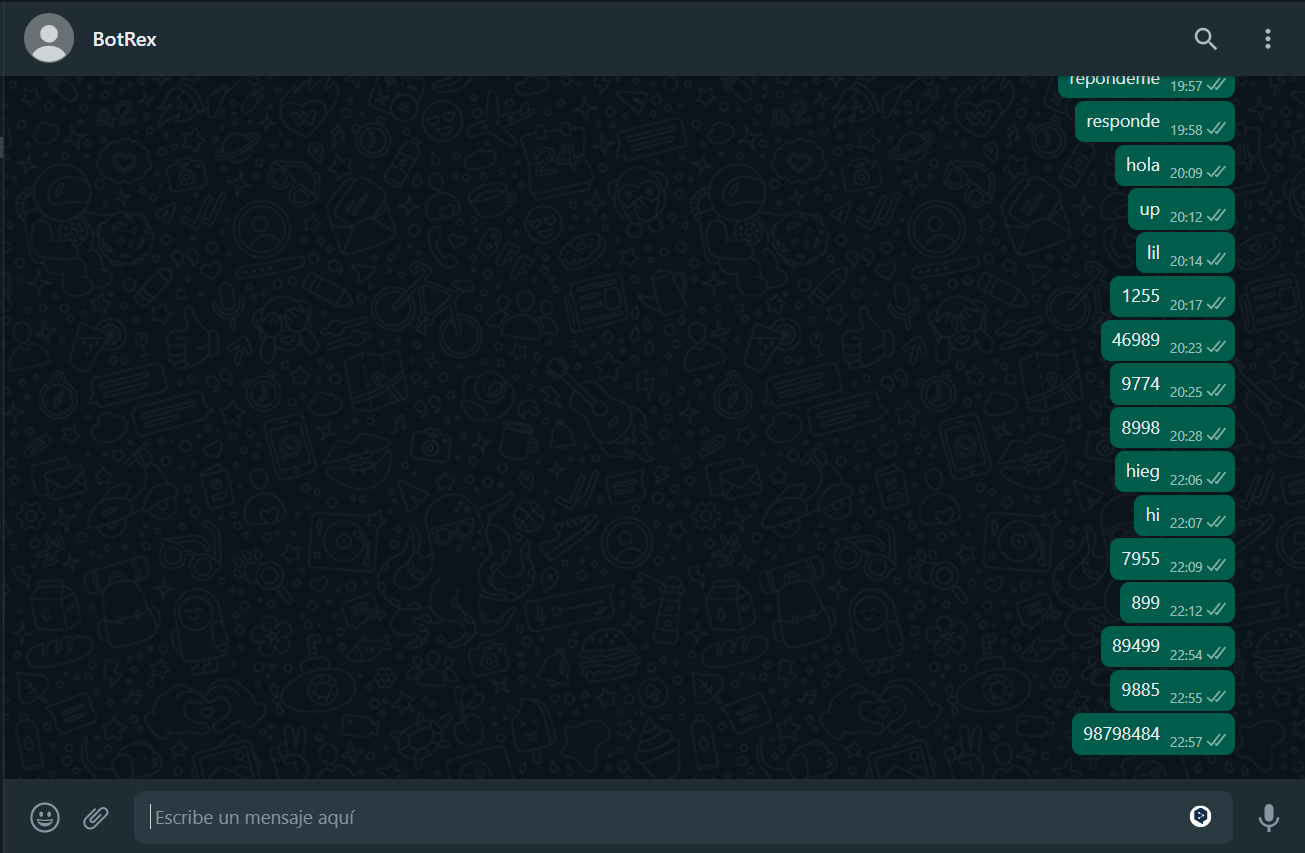